Authentication
Authentication
By Default, we are using Firebase for auth, if you want to change it to Jwt for that follow below steps.
1. Jwt Method
// -----------------------------------------------------------------------------------------
// File : src/index.tsx
// -----------------------------------------------------------------------------------------
import { AuthProvider } from 'src/guards/jwt/JwtContext'; // change is here
const root = ReactDOM.createRoot(document.getElementById('root') as HTMLElement);
root.render(
<Provider store={store}>
<Suspense fallback={<Spinner />}>
<BrowserRouter>
<AuthProvider>
<App />
</AuthProvider>
</BrowserRouter>
</Suspense>
</Provider>,
);
// -----------------------------------------------------------------------------------------
// File : src/guards/authGuard/UseAuth.tsx
// -----------------------------------------------------------------------------------------
import { useContext } from 'react';
import { AuthContext } from '../jwt/JwtContext';
const useAuth = () => useContext(AuthContext);
export default useAuth;
2. Firebase Method
For firebase, incipiently change in index.tsx file as per below mention.For more details about Firebase: https://firebase.google.com/
// -----------------------------------------------------------------------------------------
// File : src/index.tsx
// -----------------------------------------------------------------------------------------
import { AuthProvider } from 'src/guards/firebase/FirebaseContext'; // change is here
const root = ReactDOM.createRoot(document.getElementById('root') as HTMLElement);
root.render(
<Provider store={store}>
<Suspense fallback={<Spinner />}>
<BrowserRouter>
<AuthProvider>
<App />
</AuthProvider>
</BrowserRouter>
</Suspense>
</Provider>,
);
// -----------------------------------------------------------------------------------------
// File : src/guards/authGuard/UseAuth.tsx
// -----------------------------------------------------------------------------------------
import { useContext } from 'react';
import AuthContext from '../firebase/FirebaseContext';
const useAuth = () => useContext(AuthContext);
export default useAuth;
Steps for Generate key
- click on “Go to console” display on the right side on firebase account.
- Then after Click on add Project.
- After Display popup menu, Enter Project name, Select Country And Click on Terms and condition Checkbox.Click on Create Project Button.
- Now, Display The Firebase Admin panel
- In Left Panel, Click on Develop option. Now Click on authentication link.
- Click on the “Web Setup” button.
- Now, copy the configuration and change in Flexy-react project in ‘src/components/Firebase/firebase.js’.
- Now go to sign-in Method tab.Enable Email/Password and Anonymous in sign-in providers.
- Now, Click on database link from left Panel.
- Click on the create a database button.
- Display Popup in Select “start in locked mode” and Click on Enable Button.
- Now, select real-time Database.
- Click on rules tab. change the read and write rules from false to true.
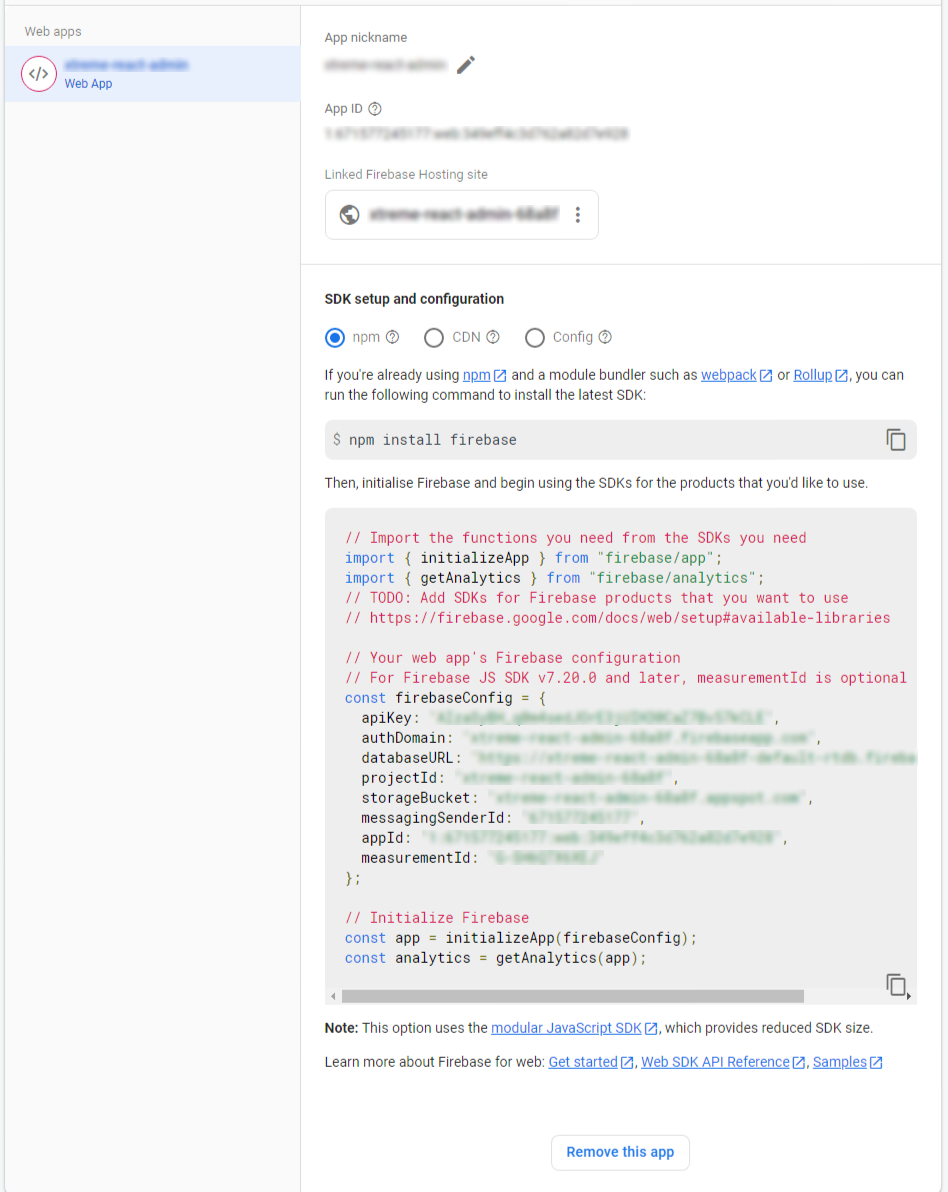
How to add key into template ?
// -----------------------------------------------------------------------------------------
// File: src/guards/firebase/Firebase.tsx
// -----------------------------------------------------------------------------------------
const firebaseConfig = {
apiKey: 'API_KEY',
authDomain: 'AUTH_DOMAIN',
projectId: 'PROJECT_ID',
storageBucket: 'STORAGE_BUCKET',
messagingSenderId: 'SENDER_ID',
appId: 'API_ID',
measurementId: 'M_ID',
databaseURL: 'DATABASE_URL',
};