Theme Settings
Customizer Usage
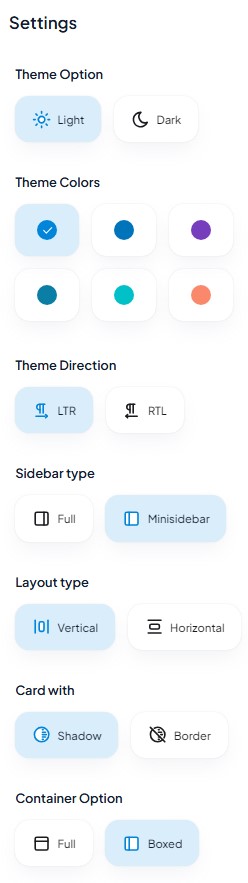
The Customizer enables you and your customers to modify the primary colors, themes, skins, layout options, and overall appearance of the template in real time.
While we recommend using this for enhanced customization, its use is entirely optional. Please review both scenarios carefully to understand its usage and benefits.
You must have below files in your project :
1. config.ts Path: /src/app/context/config.ts
2. CustomizerContext.tsx Path: /src/app/context/customizerContext.tsx
3. Customizer.tsx Path: /src/app/(DashboardLayout)/layout/shared/customizer/Customizer.tsx
1. How to set default settings ?
// ----------------------------------------------------
// File:src/context/CustomizerContext.tsx
// ----------------------------------------------------
const initialState = {
activeDir: 'ltr',
activeMode: 'light', // This can be light or dark
activeTheme: 'BLUE_THEME', // BLUE_THEME, AQUA_THEME, GREEN_THEME, PURPLE_THEME, ORANGE_THEME, CYAN_THEME
SidebarWidth: 270,
MiniSidebarWidth: 87,
TopbarHeight: 70,
isLayout: 'boxed', // This can be full or boxed
isCollapse: false, // to make sidebar Mini by default
isSidebarHover: false,
isMobileSidebar: false,
isHorizontal: false,
isLanguage: 'en',
isCardShadow: true,
borderRadius: 7,
};
2. How to set right-to-left (RTL) settings ?
// ----------------------------------------------------
// File:src/context/CustomizerContext.tsx
// ----------------------------------------------------
const initialState = {
activeDir: 'rtl', // Change is here
activeMode: 'light',
activeTheme: 'BLUE_THEME',
SidebarWidth: 270,
MiniSidebarWidth: 87,
TopbarHeight: 70,
isLayout: 'boxed',
isCollapse: false,
isSidebarHover: false,
isMobileSidebar: false,
isHorizontal: false,
isLanguage: 'en',
isCardShadow: true,
borderRadius: 7,
};
3. How to set Dark theme ?
// ----------------------------------------------------
// File:src/context/CustomizerContext.tsx
// ----------------------------------------------------
const initialState = {
activeDir: 'ltr',
activeMode: 'dark', // Change is here
activeTheme: 'BLUE_THEME',
SidebarWidth: 270,
MiniSidebarWidth: 87,
TopbarHeight: 70,
isLayout: 'boxed',
isCollapse: false,
isSidebarHover: false,
isMobileSidebar: false,
isHorizontal: false,
isLanguage: 'en',
isCardShadow: true,
borderRadius: 7,
};
4. How to change color theme ?
// ----------------------------------------------------
// File:src/context/CustomizerContext.tsx
// ----------------------------------------------------
const initialState = {
activeDir: 'ltr',
activeMode: 'light',
// BLUE_THEME, AQUA_THEME, GREEN_THEME, PURPLE_THEME, ORANGE_THEME, CYAN_THEME
activeTheme: 'AQUA_THEME', // Change is here // You can use any of above theme
SidebarWidth: 270,
MiniSidebarWidth: 87,
TopbarHeight: 70,
isLayout: 'boxed',
isCollapse: false,
isSidebarHover: false,
isMobileSidebar: false,
isHorizontal: false,
isLanguage: 'en',
isCardShadow: true,
borderRadius: 7,
};
5. How to set Horizontal layout ?
// ----------------------------------------------------
// File:src/context/CustomizerContext.tsx
// ----------------------------------------------------
const initialState = {
activeDir: 'ltr',
activeMode: 'light',
activeTheme: 'BLUE_THEME',
SidebarWidth: 270,
MiniSidebarWidth: 87,
TopbarHeight: 70,
isLayout: 'boxed',
isCollapse: false,
isSidebarHover: false,
isMobileSidebar: false,
isHorizontal: true, // Change is here
isLanguage: 'en',
isCardShadow: true,
borderRadius: 7,
};
6. How to set Fullwidth layout ?
// ----------------------------------------------------
// File:src/context/CustomizerContext.tsx
// ----------------------------------------------------
const initialState = {
activeDir: 'ltr',
activeMode: 'light',
activeTheme: 'BLUE_THEME',
SidebarWidth: 270,
MiniSidebarWidth: 87,
TopbarHeight: 70,
isLayout: 'full', // Change is here // 'full / boxed'
isCollapse: false,
isSidebarHover: false,
isMobileSidebar: false,
isHorizontal: false,
isLanguage: 'en',
isCardShadow: true,
borderRadius: 7,
};
7. How to set Boxed layout ?
// ----------------------------------------------------
// File:src/context/CustomizerContext.tsx
// ----------------------------------------------------
const initialState = {
activeDir: 'ltr',
activeMode: 'light',
activeTheme: 'BLUE_THEME',
SidebarWidth: 270,
MiniSidebarWidth: 87,
TopbarHeight: 70,
isLayout: 'boxed', // Change is here // 'full / boxed'
isCollapse: false,
isSidebarHover: false,
isMobileSidebar: false,
isHorizontal: false,
isLanguage: 'en',
isCardShadow: true,
borderRadius: 7,
};
8. How to set Minisidebar ?
// ----------------------------------------------------
// File:src/context/CustomizerContext.tsx
// ----------------------------------------------------
const initialState = {
activeDir: 'ltr',
activeMode: 'light',
activeTheme: 'BLUE_THEME',
SidebarWidth: 270,
MiniSidebarWidth: 87,
TopbarHeight: 70,
isLayout: 'boxed',
isCollapse: true, // Change is here
isSidebarHover: false,
isMobileSidebar: false,
isHorizontal: false,
isLanguage: 'en',
isCardShadow: true,
borderRadius: 7,
};
9. How to set card with shadow ?
// ----------------------------------------------------
// File:src/context/CustomizerContext.tsx
// ----------------------------------------------------
const initialState = {
activeDir: 'ltr',
activeMode: 'light',
activeTheme: 'BLUE_THEME',
SidebarWidth: 270,
MiniSidebarWidth: 87,
TopbarHeight: 70,
isLayout: 'boxed',
isCollapse: false,
isSidebarHover: false,
isMobileSidebar: false,
isHorizontal: false,
isLanguage: 'en',
isCardShadow: true, // Change is here
borderRadius: 7,
};
10. How to set card with border ?
// ----------------------------------------------------
// File:src/context/CustomizerContext.tsx
// ----------------------------------------------------
const initialState = {
activeDir: 'ltr',
activeMode: 'light',
activeTheme: 'BLUE_THEME',
SidebarWidth: 270,
MiniSidebarWidth: 87,
TopbarHeight: 70,
isLayout: 'boxed',
isCollapse: false,
isSidebarHover: false,
isMobileSidebar: false,
isHorizontal: false,
isLanguage: 'en',
isCardShadow: false, // Change is here
borderRadius: 7,
};
11. How to set theme border radius ?
// ----------------------------------------------------
// File:src/context/CustomizerContext.tsx
// ----------------------------------------------------
const initialState = {
activeDir: 'ltr',
activeMode: 'light',
activeTheme: 'BLUE_THEME',
SidebarWidth: 270,
MiniSidebarWidth: 87,
TopbarHeight: 70,
isLayout: 'boxed',
isCollapse: false,
isSidebarHover: false,
isMobileSidebar: false,
isHorizontal: false,
isLanguage: 'en',
isCardShadow: true,
borderRadius: 10, // Change is here
};