Authentication
By Default, we are using NextAuth for auth, if you want to change it to Firebase or Supabase for that follow below steps.
Select the NextAuth Demo folder
//packages/typescript/NextAuth
npm install
Global App Setup with Authentication, Theme, and RTL
// -----------------------------------------------------------------------------------------
// File : packages/typescript/NextAuth/src/app/app.tsx
// -----------------------------------------------------------------------------------------
import { AuthProvider } from 'src/app/context/AuthContext';
const MyApp = ({ children }) => {
return (
<SessionProvider session={session}>
<AuthProvider> //... Setting up Auth Context Provider for NextAuth, Firebase, and Supabase
<AppRouterCacheProvider options={{enableCssLayer:true }}>
<ThemeProvider theme={theme}>
<RTL direction={activeDir}>
{children}
</RTL>
</ThemeProvider>
</AppRouterCacheProvider>
</AuthProvider>
</SessionProvider>
)
};
1. NextAuth
Folders And Files
Inside that folder you will get this file src > app > api > auth > [...nextauth] > route.js
//src > app > api > auth > [...nextauth] > route.js
import NextAuth from "next-auth"
import GoogleProvider from 'next-auth/providers/google';
const handler = NextAuth({
// ... your change will be here
providers: [
GoogleProvider({
clientId: process.env.GOOGLE_CLIENT_ID,
clientSecret: process.env.GOOGLE_CLIENT_SECRET,
})
]
})
export { handler as GET, handler as POST }
Setup Environment Variables
// this is your .env file
GOOGLE_CLIENT_ID="Your Id"
GOOGLE_CLIENT_SECRET="Your GOOGLE_CLIENT_SECRET Key"
NEXTAUTH_SECRET="Your NEXTAUTH_SECRET Key"
NEXTAUTH_URL="http://localhost:3000/"
GITHUB_ID="Your GITHUB_ID"
GITHUB_SECRET="your GITHUB_SECRET"
Platform Configuration
// -----------------------------------------------------------------------------------------
// File : src/app/context/AuthContext.tsx
// -----------------------------------------------------------------------------------------
const initialState : InitialStateType = {
platform: 'NextAuth', //Change platform to NextAuth for NextAuth Authentication
}
How To get Google Client Id and Secret Key ?
1) Go to https://console.cloud.google.com/getting-started
2) Checkout the video : Watch
Video
3) You can create Github id and secret key and replace in .env file. (follow this step to create: https://authjs.dev/guides/configuring-github)
4) Setup callback url http://localhost:3000/api/auth/callback/github while creating a id and key
5) Once you replace the id and secret key you just need to test the sign in page.
6) You can add any provider from this link : https://next-auth.js.org/providers/ you just need to add those into router.js/ts file
How To restrict any page if user is not signed in ?
curerently we targeted dashboard pages to restrict user
//just import this
import { useSession, signIn, signOut } from "next-auth/react"
export default function YourConst() {
// extracting data from usesession as session
const { data: session } = useSession()
// checking if sessions exists
if (session) {
// rendering components for logged in users
return (...your html)
}
return(...else html)
}
2. Firebase Method
For more details about Firebase: https://firebase.google.com/
Folders And Files
Inside that folder you will get this file src > guards > authGuard > UseAuth
// -----------------------------------------------------------------------------------------
// File : src/guards/authGuard/UseAuth.tsx
// -----------------------------------------------------------------------------------------
import { useContext } from 'react';
import AuthContext from 'src/app/context/AuthContext';
const useAuth = () => useContext(AuthContext);
export default useAuth;
Platform Configuration
// -----------------------------------------------------------------------------------------
// File : src/app/context/AuthContext.tsx
// -----------------------------------------------------------------------------------------
const initialState : InitialStateType = {
platform: 'Firebase', //Change platform to Firebase for Firebase Authentication
}
Steps for Generate key
- click on “Go to console” display on the right side on firebase account.
- Then after Click on add Project.
- After Display popup menu, Enter Project name, Select Country And Click on Terms and condition Checkbox.Click on Create Project Button.
- Now, Display The Firebase Admin panel
- In Left Panel, Click on Develop option. Now Click on authentication link.
- Click on the “Web Setup” button.
- Now, copy the configuration and change in MaterialM Nextjs project in ‘src/components/Firebase/firebase.ts’.
- Now go to sign-in Method tab.Enable Email/Password and Anonymous in sign-in providers.
- Now, Click on database link from left Panel.
- Click on the create a database button.
- Display Popup in Select “start in locked mode” and Click on Enable Button.
- Now, select real-time Database.
- Click on rules tab. change the read and write rules from false to true.
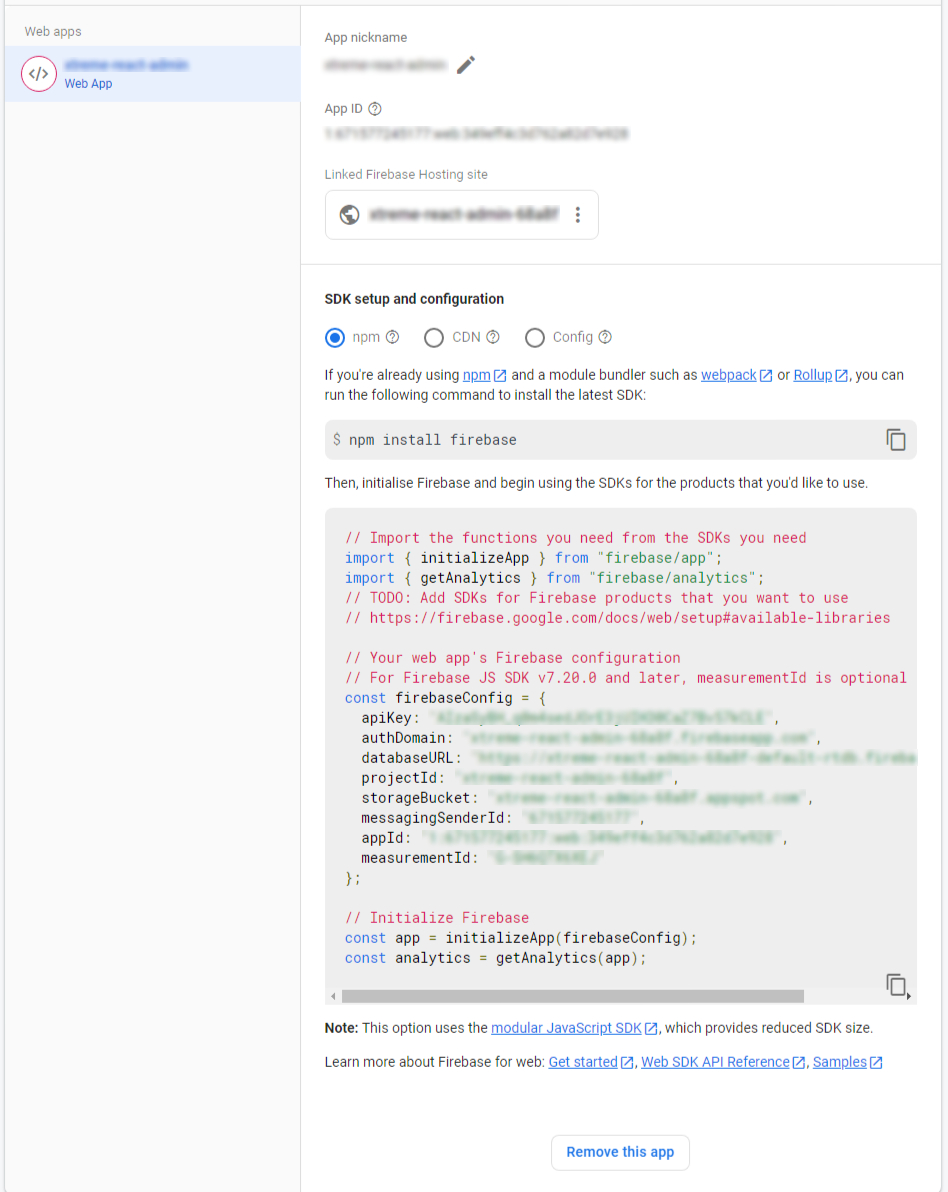
How to add key into template ?
// -----------------------------------------------------------------------------------------
// File: src/app/guards/firebase/Firebase.tsx
// -----------------------------------------------------------------------------------------
const firebaseConfig = {
apiKey: 'API_KEY',
authDomain: 'AUTH_DOMAIN',
projectId: 'PROJECT_ID',
storageBucket: 'STORAGE_BUCKET',
messagingSenderId: 'SENDER_ID',
appId: 'API_ID',
databaseURL: 'DATABASE_URL',
};
3. Supabase Method
Folders And Files
Create .env file
// this is your .env file
NEXT_PUBLIC_SUPABASE_URL="Your Url"
NEXT_PUBLIC_SUPABASE_ANON_KEY="Your SUPABASE_ANON_KEY Key"
Inside that folder you will get this file src > app > guards > supabase > supabaseClient.ts
import {createClient} from "@supabase/supabase-js"
const supabaseUrl: string | any =process.env.NEXT_PUBLIC_SUPABASE_URL;
const supabaseKey: string | any =process.env.NEXT_PUBLIC_SUPABASE_ANON_KEY;
export const supabase = createClient(supabaseUrl,supabaseKey);
Platform Configuration
// -----------------------------------------------------------------------------------------
// File : src/app/context/AuthContext.tsx
// -----------------------------------------------------------------------------------------
const initialState : InitialStateType = {
platform: 'Supabase', //Change platform to Supabase for Supabase Authentication
}
Steps for Generate key
- Go to “https://supabase.com/
- click on "Sign Up" or "Log In" and complete the process.
- On the dashboard, click on the "New Project" button.
- After Display popup menu, Enter Project name, Select Country.Click on Create Project Button.
- Once your project is created, go to the Project Dashboard.
- In Left Panel, Now Click on authentication Tab.
- By default, Email & Password authentication is enabled, which allows users to sign up and sign in using their email and password.
- You can also enable OAuth Providers like Google, GitHub, Twitter, etc.
- In the Settings tab under Authentication, you can customize email templates for user verification, password reset emails, and more.
- Now, From the left sidebar, click on API.
- Poject Url: This is used for client-side authentication, to interact with your Supabase project. Copy this URL.
- Anon Key: This is used for client-side authentication, Copy this key.
- Install Supabase : npm install @supabase/supabase-js
- Create an .env File to Store Your Keys
// this is your .env file
NEXT_PUBLIC_SUPABASE_URL="Your Url"
NEXT_PUBLIC_SUPABASE_ANON_KEY="Your SUPABASE_ANON_KEY Key"
.png)
import {createClient} from "@supabase/supabase-js"
const supabaseUrl: string | any =process.env.NEXT_PUBLIC_SUPABASE_URL;
const supabaseKey: string | any =process.env.NEXT_PUBLIC_SUPABASE_ANON_KEY;
export const supabase = createClient(supabaseUrl,supabaseKey);