Theme Settings
Customizer Usage
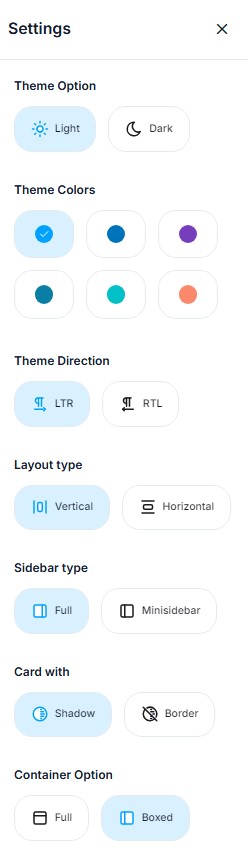
The Customizer enables you and your customers to modify the primary colors, themes, skins, layout options, and overall appearance of the template in real time.
While we recommend using this for enhanced customization, its use is entirely optional. Please review both scenarios carefully to understand its usage and benefits.
You must have below files in your project :
1. config.ts Path: /src/app/context/config.ts
2. CustomizerContext.tsx Path: /src/app/context/CustomizerContext/index.tsx
3. Customizer.tsx Path: /src/app/(DashboardLayout)/layout/shared/customizer/Customizer.tsx
1. How to set default settings ?
// ----------------------------------------------------
// File: src/app/context/config.ts
// ----------------------------------------------------
const config = {
activeDir: "ltr", // This can be ltr or rtl
activeMode: "light", // This can be light or dark
activeTheme: "BLUE_THEME", // BLUE_THEME, GREEN_THEME, AQUA_THEME, PURPLE_THEME, ORANGE_THEME
activeLayout: "vertical", // This can be vertical or horizontal
isLayout: "boxed", // This can be full or boxed
isSidebarHover: false,
isCollapse:"full-sidebar",
isLanguage: "en",
isCardShadow: true,
isMobileSidebar: false,
isHorizontal: false,
isBorderRadius: 24,
sidebarWidth: 320,
miniSidebarWidth: 87,
topbarHeight: 70,
};
2. How to set right-to-left (RTL) settings ?
// ----------------------------------------------------
// File: src/app/context/config.ts
// ----------------------------------------------------
const config = {
activeDir: "rtl", // change is here
activeMode: "light",
activeTheme: "BLUE_THEME",
activeLayout: "vertical",
isLayout: "boxed",
isSidebarHover: false,
isCollapse:"full-sidebar",
isLanguage: "en",
isCardShadow: true,
isMobileSidebar: false,
isHorizontal: false,
isBorderRadius: 24,
sidebarWidth: 320,
miniSidebarWidth: 87,
topbarHeight: 70,
};
3. How to set Dark theme ?
// ----------------------------------------------------
// File: src/app/context/config.ts
// ----------------------------------------------------
const config = {
activeDir: "ltr",
activeMode: "dark", // change is here
activeTheme: "BLUE_THEME",
activeLayout: "vertical",
isLayout: "boxed",
isSidebarHover: false,
isCollapse:"full-sidebar",
isLanguage: "en",
isCardShadow: true,
isMobileSidebar: false,
isHorizontal: false,
isBorderRadius: 24,
sidebarWidth: 320,
miniSidebarWidth: 87,
topbarHeight: 70,
};
4. How to change color theme ?
// ----------------------------------------------------
// File: src/app/context/config.ts
// ----------------------------------------------------
const config = {
activeDir: "ltr",
activeMode: "light",
activeTheme: "GREEN_THEME", // change is here // BLUE_THEME, GREEN_THEME, AQUA_THEME, PURPLE_THEME, ORANGE_THEME
activeLayout: "vertical",
isLayout: "boxed",
isSidebarHover: false,
isCollapse:"full-sidebar",
isLanguage: "en",
isCardShadow: true,
isMobileSidebar: false,
isHorizontal: false,
isBorderRadius: 24,
sidebarWidth: 320,
miniSidebarWidth: 87,
topbarHeight: 70,
};
5. How to set Horizontal layout ?
// ----------------------------------------------------
// File: src/app/context/config.ts
// ----------------------------------------------------
const config = {
activeDir: "ltr",
activeMode: "light",
activeTheme: "BLUE_THEME",
activeLayout: "horizontal", // change is here
isLayout: "boxed",
isSidebarHover: false,
isCollapse:"full-sidebar",
isLanguage: "en",
isCardShadow: true,
isMobileSidebar: false,
isHorizontal: false,
isBorderRadius: 24,
sidebarWidth: 320,
miniSidebarWidth: 87,
topbarHeight: 70,
};
6. How to set Fullwidth layout ?
// ----------------------------------------------------
// File: src/app/context/config.ts
// ----------------------------------------------------
const config = {
activeDir: "ltr",
activeMode: "light",
activeTheme: "BLUE_THEME",
activeLayout: "vertical",
isLayout: "full", // change is here
isSidebarHover: false,
isCollapse:"full-sidebar",
isLanguage: "en",
isCardShadow: true,
isMobileSidebar: false,
isHorizontal: false,
isBorderRadius: 24,
sidebarWidth: 320,
miniSidebarWidth: 87,
topbarHeight: 70,
};
7. How to set Boxed layout ?
const config = {
activeDir: "ltr",
activeMode: "light",
activeTheme: "BLUE_THEME",
activeLayout: "vertical",
isLayout: "boxed", // change is here
isSidebarHover: false,
isCollapse:"full-sidebar",
isLanguage: "en",
isCardShadow: true,
isMobileSidebar: false,
isHorizontal: false,
isBorderRadius: 24,
sidebarWidth: 320,
miniSidebarWidth: 87,
topbarHeight: 70,
};
8. How to set Minisidebar ?
// ----------------------------------------------------
// File: src/app/context/config.ts
// ----------------------------------------------------
const config = {
activeDir: "ltr",
activeMode: "light",
activeTheme: "BLUE_THEME",
activeLayout: "vertical",
isLayout: "boxed",
isSidebarHover: false,
isCollapse:"mini-sidebar", // change is here
isLanguage: "en",
isCardShadow: true,
isMobileSidebar: false,
isHorizontal: false,
isBorderRadius: 24,
sidebarWidth: 320,
miniSidebarWidth: 87,
topbarHeight: 70,
};
9. How to set card with shadow ?
// ----------------------------------------------------
// File: src/app/context/config.ts
// ----------------------------------------------------
const config = {
activeDir: "ltr",
activeMode: "light",
activeTheme: "BLUE_THEME",
activeLayout: "vertical",
isLayout: "boxed",
isSidebarHover: false,
isCollapse:"full-sidebar",
isLanguage: "en",
isCardShadow: true, // change is here
isMobileSidebar: false,
isHorizontal: false,
isBorderRadius: 24,
sidebarWidth: 320,
miniSidebarWidth: 87,
topbarHeight: 70,
};
10. How to set card with border ?
// ----------------------------------------------------
// File: src/app/context/config.ts
// ----------------------------------------------------
const config = {
activeDir: "ltr",
activeMode: "light",
activeTheme: "BLUE_THEME",
activeLayout: "vertical",
isLayout: "boxed",
isSidebarHover: false,
isCollapse:"full-sidebar",
isLanguage: "en",
isCardShadow: false, // change is here
isMobileSidebar: false,
isHorizontal: false,
isBorderRadius: 24,
sidebarWidth: 320,
miniSidebarWidth: 87,
topbarHeight: 70,
};
11. How to set theme border radius ?
// ----------------------------------------------------
// File: src/app/context/config.ts
// ----------------------------------------------------
const config = {
activeDir: "ltr",
activeMode: "light",
activeTheme: "BLUE_THEME",
activeLayout: "vertical",
isLayout: "boxed",
isSidebarHover: false,
isCollapse:"full-sidebar",
isLanguage: "en",
isCardShadow: true,
isMobileSidebar: false,
isHorizontal: false,
isBorderRadius: 24, // change is here
sidebarWidth: 320,
miniSidebarWidth: 87,
topbarHeight: 70,
};